일렉트론이란?
일렉트론은 HTML, CSS, javascript를 사용하여 데스크톱 애플리케이션을 빌드하는 프레임워크입니다.
프론트엔드 개발자라면 당연히 잘 다뤄야할 언어로 데스크톱 어플리케이션도 만들 수 있으니 프론트엔드 개발자에겐 매우 유리합니다. Jquery, React, Angular 등 웹 프론트 개발에 씌였던 라이브러리들을 사용할 수 있기 때문입니다.
대표적인 일렉트론으로 개발한 프로그램으로는 Discord, Slack, VSCode 등이 있습니다.
웹 개발용 언어가 데스크탑 애플리케이션에서 작동할 수 있는 이유는 크롬의 오픈소스 버전인 크로미움을 사용했기 때문입니다. 크로미움과 Node.js를 각각 웹에서 프론트엔드와 백엔드의 역할을 해줍니다. 또한 크로스플랫폼이 가능한 이유이기도 합니다.
일렉트론 준비하기
1. Node.js의 최신 LTS버전을 설치합니다
Node.js 설치
2. npm init과 electron을 설치합니다
mkdir my-electron-app && cd my-electron-app
npm init
npm install --save-dev electron
3. main.js 파일을 작성합니다
const {app, BrowserWindow} = require('electron')
let mainWindow
function createWindow () {
mainWindow = new BrowserWindow({
width: 1000, height: 800,
webPreferences: {
contextIsolation: false,
nodeIntegration: true
}
})
mainWindow.loadFile('index.html')
mainWindow.webContents.openDevTools();
mainWindow.on('closed', () => {
mainWindow = null
})
}
app.on('ready', createWindow)
app.on('window-all-closed', () => {
if (process.platform !== 'darwin') app.quit()
})
app.on('activate', () => {
if (mainWindow === null) createWindow()
})
4. preload.js를 작성합니다
// preload.js
// All the Node.js APIs are available in the preload process.
// It has the same sandbox as a Chrome extension.
window.addEventListener('DOMContentLoaded', () => {
const replaceText = (selector, text) => {
const element = document.getElementById(selector)
if (element) element.innerText = text
}
for (const dependency of ['chrome', 'node', 'electron']) {
replaceText(`${dependency}-version`, process.versions[dependency])
}
})
5. index.html을 작성합니다
<!--index.html-->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<!-- https://developer.mozilla.org/en-US/docs/Web/HTTP/CSP -->
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self'">
<title>Hello World!</title>
</head>
<body>
<h1>Hello World!</h1>
We are using Node.js <span id="node-version"></span>,
Chromium <span id="chrome-version"></span>,
and Electron <span id="electron-version"></span>.
<!-- You can also require other files to run in this process -->
<script src="./renderer.js"></script>
</body>
</html>
6. package.json에 start 스크립트를 추가합니다
{
"name": "electron",
"version": "1.0.0",
"description": "",
"main": "main.js",
"scripts": {
"start": "electron ."
},
"author": "",
"license": "ISC",
"devDependencies": {
"electron": "^27.0.2"
}
}
7. npm start를 입력하여 애플리케이션을 실행합니다
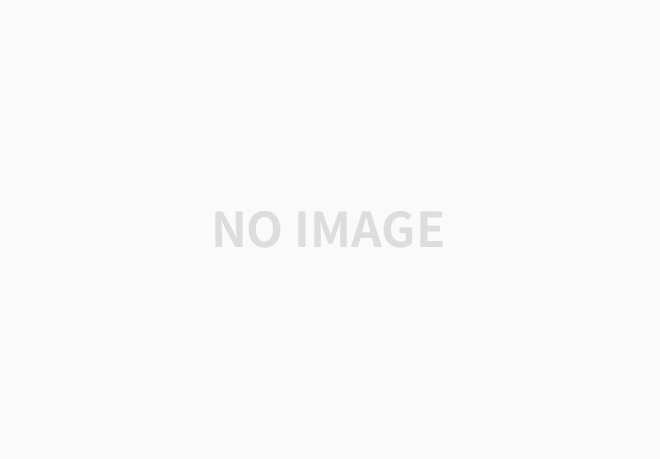
참고
댓글